The Sass Way!
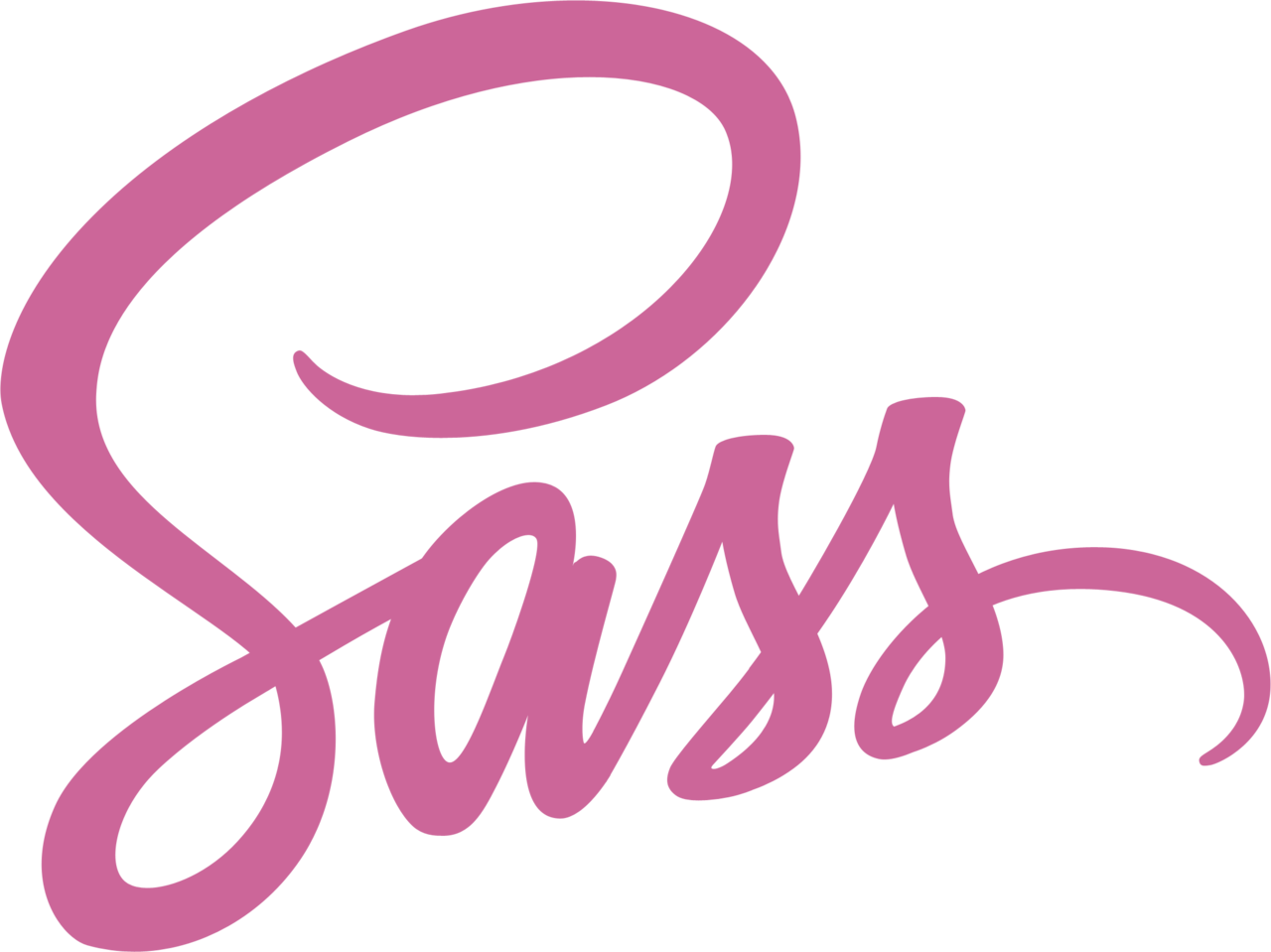
If you get frustrated with how messy and unmanageable your CSS gets, wish you didn’t have to specify properties over and over, or wished you could compute mathematical operations then you need Sass an extension of CSS that does all of this and more. I wished I had known about Sass sooner as I would have saved countless hours of time writing CSS. Lucky for you today I am going to give you the full crash course of how to write in the Sass scripting language. Don’t worry its as easy as learning Texas Holdem and you will thank yourself for learning it.
What is Sass?
Well according to the official Sass site Sass is:
CSS with superpowers
Sass is a preprocessed language written in Ruby that extends the functionality of css; preprocessed meaning compiler must compile the code from sass to css.
Below is a list of powers you get:
- Variables
- Nesting css inside other css selectors
- Partials
- Extend classes - allow classes to be used inside other classes
- Math operations and conditionals
- Mixins - aka functions/methods
There are two different versions of Sass the old format ends in extentions .sass
and the new format ends in .scss
I will be teaching you the .scss
way.
Variables
Variables are great to store property values so you don’t have to keep referencing them over again; colors are a great use for this. To create a variable begin the variable name with a $
sign and then the variable name followed by a colon and the value $varname: value
. To use the the variable simply reference the variable name after the css property. Another great use is to store font list to a variable. See below for examples
// variables in sass start off with at $variableName: value
$offwhite: #EEE86D;
body { background-color: $offwhite }
// you can even pair selector and value as variables
$color-main: $offwhite
body { background-color: $color-main; }
// Variables are really useful when using them for fonts
$font-main: 'Oswald', 'Helvetica', 'serif';
Nesting
One of the best features of Sass is the ability to nest slectors. This helps writting less code and makes it more readable and organized. Think about if you had a div
and inside was an ul
, li
and inside the list was an anchor ‘a’. Instead of having to traverse each selector individually I can nest inside the div and edit each selector’s properties; basically creating sub rules. Nest only a few levels deep otherwise it may be hard to manage. See below.
// putting rules inside other rules creates nested sub rules
.div {
margin: 0;
padding: 0;
ul {
list-style-type: none;
border-top: 1px dotted $color-item-border;
.list {
display: inline-block;
}
}
}
Paritals
Just like you do when you develop, creating partials is a great way to split up sections of your css and making it easier to pinpoint parts of your css. When creating a a partial you begin the file name with an underscore; for example, _variables
will house all your variables. You can seperate more than your variables such as mixins, your entire footer or header css into partials. To import a partial into another file use the @import
keyword followed by the filename; `@import ‘header;’.
// to import files partial files use @import
// you could have all of these imported in style.scss file
// partial file begin with _ ex: _variables
@import 'variables';
@import 'base';
@import 'modules/media';
Mixins
Mixins are just like functions that you see in javascript. It helps keep your code DRY (don’t repeat yourself) and becomes very useful when you have to keep repeating properties with differnt values or calculations. I can create a simple mixin using the format: @mixin mixinName($args) { code }
. To use the mixin you simple use the @import
keyword so @import mixinName($args)
. One more thing to specify a default value of an argument use the : value
symbol. See below for detailed example.
// to create a mixin use @mixin mixinName($varName) { code }
@mixin setSize($height, $width) {
height: $height;
width: $width;
}
// to use mixin - file path is relative to style.css NOT style.scss
img {
@include setSize(auto, 600px)
}
// you can specify a default value using : value
// below height value is 100% of viewport height
@mixin setSize($height: auto, $width: 100%) { code }
@extend Keyword
The @extend
keyword allows you to import another selector’s properties and values into another; its basically inhertiance the second selector inherits all the properties. To use simply write @inherit selector
.
.button {
background-color: red;
border: 1px solid blue;
color: black;
padding: 15px 32px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
}
.button-success {
@extend .button;
background-color: green;
}
Using Parent Elements With & Symbol
The &
symbol allows you to select the parent element which is really useful when applying pseudo class such as :hover :active etc…
a {
padding: 10px 5px;
&:hover {
background: $yellow;
}
}
The &
symbol is also used as a conditional if that you can apply styling to if the html doc contains the selector. See below
// you can use & for a conditional and
// below says if inside html there is a head class and typography id then apply a color of twitter blue
.title {
margin, padding: 0;
color: $color-media-head;
font-size: 2em;
#typography & {
color: $twt-clr
}
}
Math Operaters
SASS allows you the ability to create simple mathematical calculations using operators for addition +
, subtraction -
, multiplication *
, division \
and modulus %
.
@mixin setWidth($qty) {
width: 960px / $qty * 100%;
}
Creating Arrays
To create a list/array of values with a property simply add a comma and then the value. The arrays are not 0 index so the first element is index number 1. To access a specific value use the $
parenthesis ()
and inside parenthesis put the variable name and index number: $(color 2)
.
// you can create lists using commas or spaces
$font-body: Industry, Helvetica, Serif;
// you can access a specific element using $(varName index#) NOT 0 index
$font-body: Industry, Helvetica, Serif;
$(font-body 1);
If Else Conditionals
Using the @if condiiton
and @else
you can assign differnt properties and values depending on the truthiness of the condition.
// you can create lists using commas or spaces
$btn-clr: blue;
@mixin buttonBackground($btn-clr) {
@if $btn-clr == blue {
background-color: red;
} @else {
background-color: green;
}
}
Loops & Iterations
Yes, you can even loop through your variables and assign different properties for each iteration. Since there are many types of loops take a look at this great article on loops. Below is an example of a using a for loop to change the color of each heading tag.
// you can use for loop by @for counterName from start# through end# { code }
$colors: $red, $blue, $green, $orange, $yellow, $purple;
@for $item from 1 through length($colors) {
h#{$item} {
color: $(colors, $item);
}
}
Creating Hashes
Last, I want to show you how to create a hash and access a key and its value. To create a hash you use the format $variable: ( key: value, key: value)
and to access one of the values of the key you use map-get function: map-get($map, $key)
. Here is a nice detailed article on hashes.
$button-color: (
default: $white,
danger: $red,
primary: $blue,
success: $green
);
.btn-sucess { color: map-get($button-color, $success) }
Commenting
One final thing. Comments are really useful when documenting your code to understand what is executing in the below statements. Create a comment b /* comment */
. You can force a comment to show up even in minified versions using a !
after opening /*! comment */
. you can create hidden comments using //
and the comments will not show up in processed version of stylesheet